WordPress with DeepSeek API: An Experiment
This article will guide you through the process of integrating the DeepSeek API with WordPress.
Whether you’re a seasoned developer or just starting out, you’ll find valuable insights and practical steps to enhance your site’s capabilities.
From setting up the API to implementing it in your WordPress environment, we’ve got you covered.
Getting Started with DeepSeek API
Before diving into the integration, it’s essential to understand what the DeepSeek API offers.
DeepSeek provides a suite of tools for data analysis, natural language processing, and machine learning.
These tools can be leveraged to create intelligent features on your WordPress site, such as personalized content recommendations, sentiment analysis, and more.
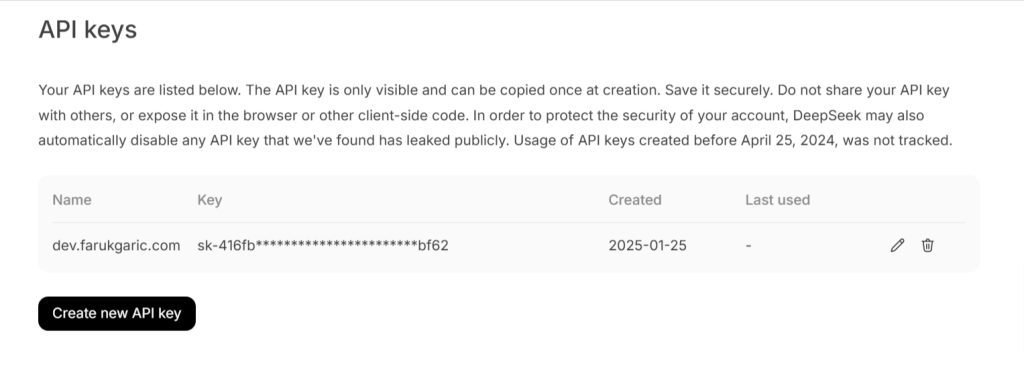
To get started, you’ll need to sign up for a DeepSeek API key. This key will authenticate your requests and allow you to access the API’s features. Once you have your API key, you can begin the integration process by installing the necessary plugins or writing custom code to connect DeepSeek with your WordPress site.
Setting Up the DeepSeek API in WordPress
When it comes to experimenting in WordPress, I find using WP CLI to be my go-to tool. Here’s the example I used while drafting this blog post.
In wp-config set the constant:
define(‘DEEPSEEK_API_KEY’, ‘your-api-key’);
<?php
/*
* @desc DeepSeek Playground
*/
class DeepSeek_Playground_Command {
/**
* Simple CMD for testing DeepSeek API
*
* ## EXAMPLES
*
* wp ds
*
* @when after_wp_load
*/
public function __invoke($args, $assoc_args) {
if (!defined('DEEPSEEK_API_KEY')) {
WP_CLI::error('Please define DEEPSEEK_API_KEY in wp-config.php');
}
$api_url = 'https://api.deepseek.com/v1/chat/completions';
$headers = [
'Content-Type' => 'application/json',
'Authorization' => 'Bearer ' . DEEPSEEK_API_KEY
];
$payload = [
'model' => 'deepseek-chat',
'messages' => [
[
'role' => 'user',
'content' => 'Help me write an article and catchy but concise title for it about using DeepSeek API with WordPress. Article content needs to have two introduction paragraphs and broken into subheadings with 2-3 paragraphs for each subtopic. Title needs to attract users to click but also not mislead and overpromise. Return format should be JSON with title and content properties where title is a string and content wrapped in proper HTML markup'
]
],
'max_tokens' => 1000
];
$response = wp_remote_post($api_url, [
'headers' => $headers,
'body' => json_encode($payload),
'timeout' => 30
]);
if (is_wp_error($response)) {
WP_CLI::error('API request failed: ' . $response->get_error_message());
}
$status_code = wp_remote_retrieve_response_code($response);
$body = json_decode(wp_remote_retrieve_body($response), true);
if ($status_code !== 200) {
WP_CLI::error("API Error [{$status_code}]: " . print_r($body, true));
}
if (!isset($body['choices'][0]['message']['content'])) {
WP_CLI::error('Unexpected response format: ' . print_r($body, true));
}
WP_CLI::success("API Response:\n" . $body['choices'][0]['message']['content']);
}
}
WP_CLI::add_command('ds', 'DeepSeek_Playground_Command');
Run the command from WP root directory: wp ds
Implementing DeepSeek Features on Your Site
With the DeepSeek API integrated into your WordPress site, the possibilities are endless.
One common use case is implementing personalized content recommendations. By analyzing user behavior and preferences, DeepSeek can suggest articles, products, or services that are most relevant to each visitor, enhancing their overall experience.
Another powerful feature is sentiment analysis. By leveraging DeepSeek’s natural language processing capabilities, you can analyze user comments, reviews, or social media posts to gauge public sentiment. This can be particularly useful for businesses looking to monitor brand reputation or gather feedback on new products.
Conclusion
Integrating the DeepSeek API with WordPress opens up a world of possibilities for enhancing your site’s functionality and user experience. From personalized content recommendations to sentiment analysis, the DeepSeek API provides the tools you need to create a more intelligent and engaging website.
By following the steps outlined in this article, you can seamlessly integrate the DeepSeek API into your WordPress site and start leveraging its powerful features. Whether you’re looking to improve user engagement, gather valuable insights, or simply stay ahead of the curve, the DeepSeek API is a valuable addition to your development toolkit.
Comments
Comments are disabled for this post