How to Add WooCommerce Custom Field to a Product page
When you need to add required input field to the product page of your WooCommerce shop and this field needs to be stored in order as an attribute and sent to the customer in confirmation email, you’ll need to add custom code to your theme’s functions.php
file. Here is the step-by-step process and the necessary code:
Step 1: Add the Input Field to the Product Page
Add the following code to your theme’s functions.php
file to display a custom input field on the product page:
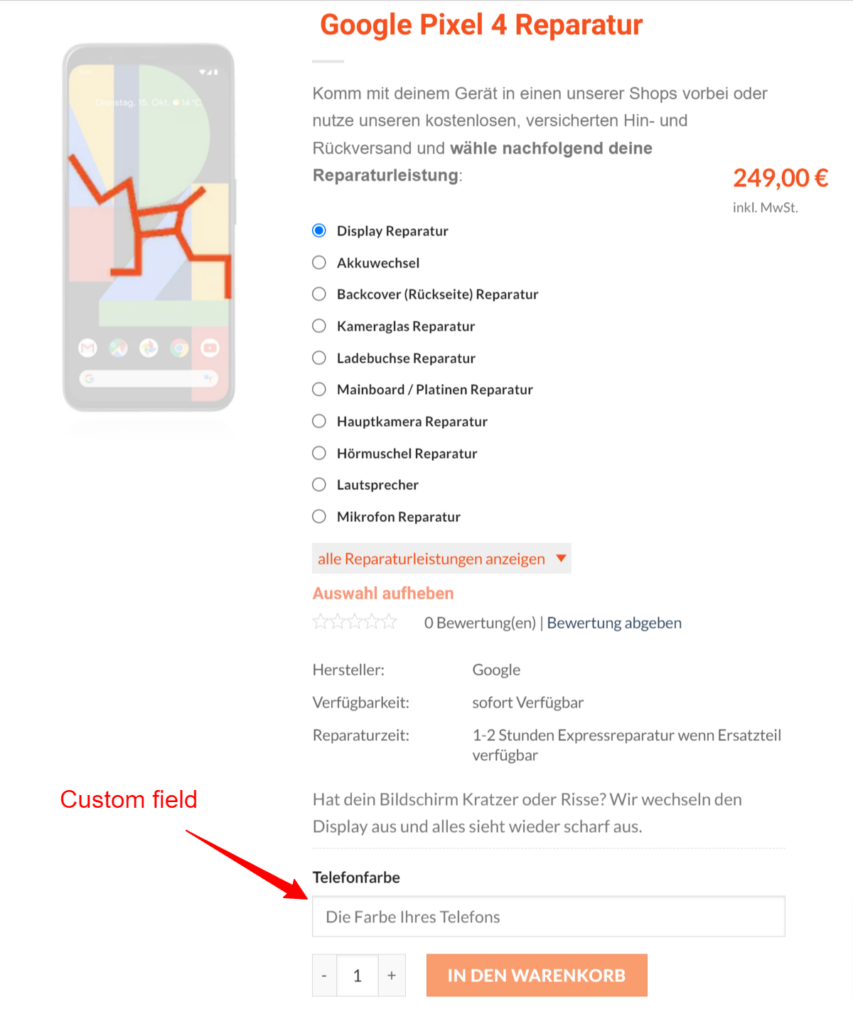
// Display the custom input field on the product page
add_action( 'woocommerce_before_add_to_cart_button', 'add_custom_field_to_product_page' );
function add_custom_field_to_product_page() {
echo '<div class="custom-field-wrapper">';
echo '<label for="custom_field">' . __( 'Custom Field', 'woocommerce' ) . '</label>';
echo '<input type="text" id="custom_field" name="custom_field" class="input-text" required />';
echo '</div>';
}
Step 2: Validate the Input Field
Ensure that the custom input field is not empty when adding a product to the cart:
// Validate the custom input field
add_filter( 'woocommerce_add_to_cart_validation', 'validate_custom_field', 10, 3 );
function validate_custom_field( $passed, $product_id, $quantity ) {
if( isset( $_POST['custom_field'] ) && empty( $_POST['custom_field'] ) ) {
wc_add_notice( __( 'Please enter a value for the custom field.', 'woocommerce' ), 'error' );
return false;
}
return $passed;
}
Step 3: Save the Custom Field Value
Save the custom input field value as an order item meta when the product is added to the cart:
// Save the custom field value to the cart item data
add_filter( 'woocommerce_add_cart_item_data', 'save_custom_field_to_cart_item', 10, 2 );
function save_custom_field_to_cart_item( $cart_item_data, $product_id ) {
if( isset( $_POST['custom_field'] ) ) {
$cart_item_data['custom_field'] = sanitize_text_field( $_POST['custom_field'] );
}
return $cart_item_data;
}
// Save the custom field value as order item meta data
add_action( 'woocommerce_add_order_item_meta', 'save_custom_field_to_order_item_meta', 10, 2 );
function save_custom_field_to_order_item_meta( $item_id, $values ) {
if ( ! empty( $values['custom_field'] ) ) {
wc_add_order_item_meta( $item_id, 'Custom Field', $values['custom_field'] );
}
}
Step 4: Display the Custom Field in Order Details
Display the custom field value in the order details on the admin side:
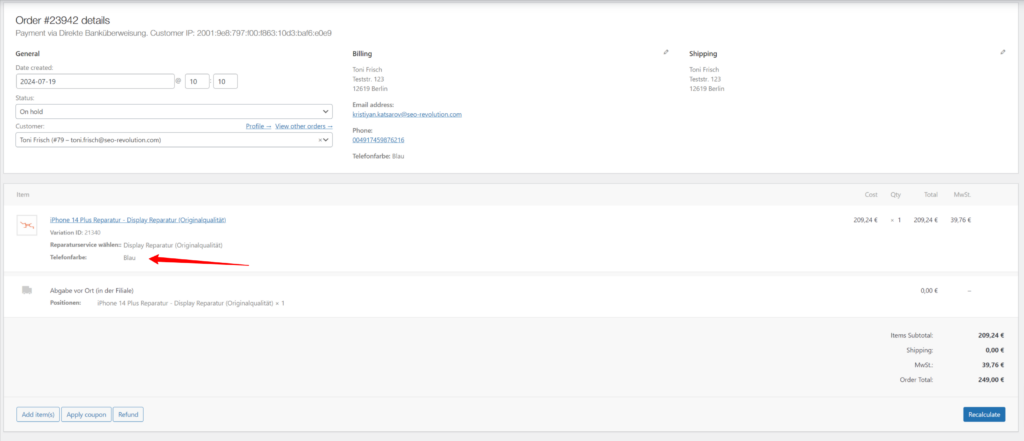
// Display the custom field value in the order admin
add_action( 'woocommerce_admin_order_data_after_billing_address', 'display_custom_field_in_admin_order_meta', 10, 1 );
function display_custom_field_in_admin_order_meta( $order ){
foreach ( $order->get_items() as $item_id => $item ) {
if ( $custom_field = wc_get_order_item_meta( $item_id, 'Custom Field', true ) ) {
echo '<p><strong>' . __( 'Custom Field', 'woocommerce' ) . ':</strong> ' . $custom_field . '</p>';
}
}
}
Step 5: Add the Custom Field to the Email
Include the custom field value in the order confirmation email:
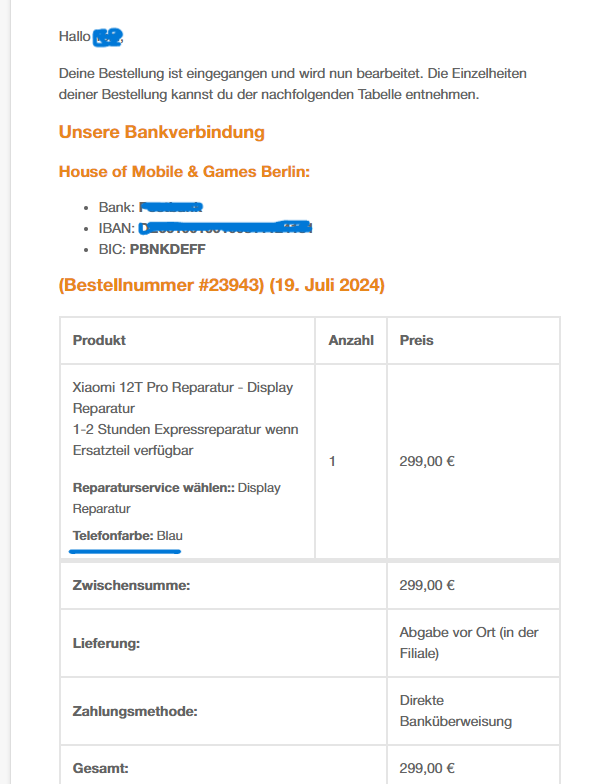
// Display the custom field value in the order email
add_filter( 'woocommerce_email_order_meta_fields', 'display_custom_field_in_order_email', 10, 3 );
function display_custom_field_in_order_email( $fields, $sent_to_admin, $order ) {
foreach ( $order->get_items() as $item_id => $item ) {
if ( $custom_field = wc_get_order_item_meta( $item_id, 'Custom Field', true ) ) {
$fields['custom_field'] = array(
'label' => __( 'Custom Field', 'woocommerce' ),
'value' => $custom_field,
);
}
}
return $fields;
}
Summary
The above code will:
- Add a custom input field to the product page.
- Validate that the input field is not empty.
- Save the custom field value in the cart and as order item meta.
- Display the custom field value in the order details in the admin.
- Include the custom field value in the order confirmation email.
Add this code to your theme’s functions.php
file and customize the field label and other details as needed.
Comments
Leave a Comment